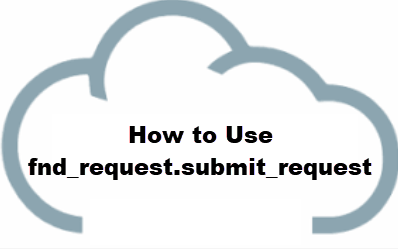
FND_REQUEST.SUBMIT_REQUEST is a PL SQL Public API of Oracle e-business suite and is used to submit concurrents programs in ORACLE Applications from the Standard Request window.
Basically when you submit a concurrent program from SRS (Standard Request Screen) internally the fnd_request.submit_request API is called which does the processing of the child programs.
So when we write our custom programs in OAF, PLSQL, FORMS, or doing some integration with Oracle EBS we may need to use the find_request API to execute the concurrent programs.
Let’s see how do we use it.
Table of Contents
FND_REQUEST.SUBMIT_REQUEST is a Function
FND_REQUEST.SUBMIT_REQUEST is a Function that returns a number after it is called. The number is the request id of the concurrent request.
If the call to FND_REQUEST.SUBMIT_REQUEST
request completes successfully, this function returns the concurrent request ID, Else, it returns 0.
Function FND_REQUEST.SUBMIT_REQUEST (application IN varchar2 default NULL, program IN varchar2 default NULL, description IN varchar2 default NULL, start_time IN varchar2 default NULL, sub_request IN boolean default FALSE argument1, argument2, ..., argument99, argument100) return number;
If you review the Database package it takes various parameters as input, these are
Application: The Application short name with which the concurrent program is registered and will be submitted.
Program – The concurrent program Short name
Description – Description of the request. This is what is displayed in SRS. This can be different for different calls and not mandatory.
Start_time – Can be a Scheduled time at which the request should start running, formatted as HH24:MI or HH24:MI:SS (Optional.)
Sub_request – Set to TRUE if the request is submitted from another parent request and should be treated as a sub-request.
Argument1…100 – Arguments for the concurrent request; up to 100 arguments are permitted. If submitting from Oracle Forms, you must specify all 100 arguments.
Example to call concurrent program from pl sql
PROCEDURE submit_standard_program(
itemtype in varchar2,
itemkey in varchar2,
actid in number,
funcmode in varchar2,
resultout in out NOCOPY varchar2) IS
ln_request_id number;
ln_inv_hold_count NUMBER:=NULL;
ln_inv_nohold_count NUMBER:=NULL;
ln_out NUMBER :=0;
TYPE type_request_table IS TABLE OF number index by binary_integer;
lr_type_request_table type_request_table;
ln_no_of_request_submitted BINARY_INTEGER:=0;
ln_count BINARY_INTEGER;
ln_interval NUMBER := 10;
ln_maxwait NUMBER :=0;
lc_request_status BOOLEAN;
lc_phase FND_LOOKUPS.MEANING%TYPE;
lc_status FND_LOOKUPS.MEANING%TYPE;
lc_dev_phase FND_LOOKUPS.MEANING%TYPE := 'START';
lc_dev_status FND_LOOKUPS.MEANING%TYPE := 'INITIAL';
lc_message FND_CONCURRENT_REQUESTS.REQ_INFORMATION%TYPE;
begin
fnd_global.apps_initialize(1068 ,56229, 724);
mo_global.init('INV');
IF ln_out=0 THEN
ln_request_id := FND_REQUEST.SUBMIT_REQUEST
(application => 'INV', -- Application Name
program => 'INCTCM', -- Program
description => 'Process transaction interface' ,
start_time => NULL, -- START_TIME
sub_request => FALSE -- SUB_REQUEST
);
-- COMMIT;
dbms_output.put_line('request id '||ln_request_id);
lr_type_request_table(ln_no_of_request_submitted):=ln_request_id;
fnd_file.put_line(fnd_file.log,ln_request_id);
ln_no_of_request_submitted:=ln_no_of_request_submitted+1;
FOR ln_count IN 0..ln_no_of_request_submitted-1
LOOP
dbms_output.put_line('request id loop '||ln_request_id);
lc_request_status := FND_CONCURRENT.WAIT_FOR_REQUEST(lr_type_request_table(ln_count),
ln_interval,
ln_maxwait,
lc_phase,
lc_status,
lc_dev_phase,
lc_dev_status,
lc_message);
END LOOP;
ELSE
FND_FILE.PUT_LINE(FND_FILE.LOG,'Invoice Validation failed');
-- retcode:=2;
END IF;
EXCEPTION
WHEN OTHERS THEN
FND_FILE.PUT_LINE(FND_FILE.LOG,'Error while posting the Concurrent Program'||SQLERRM);
end submit_standard_program;
And when you need to call with parameters
create or replace function WPSGETREQ(p_assetNumber VARCHAR2) return number is
out_reqid number;
x_tagNo VARCHAR2(300);
v_request_id NUMBER;
v_request_id2 NUMBER;
pragma autonomous_transaction;
BEGIN
begin
fnd_global.apps_initialize(1000354,52553,140);
end;
select tag_number into x_tagNo from FA_ADDITIONS_B where asset_number=p_assetNumber;
v_request_id := fnd_request.submit_request(application => 'CUSTOM', -- Application Name
program => 'WPS_LOUD', -- Program
description => 'WPS Custom Asset Report' ,
start_time => to_char(sysdate,'YYYY/MM/DD HH24:MI:SS'), -- START_TIME
sub_request => FALSE, -- SUB_REQUEST
argument1=>p_assetNumber);
v_request_id2 := fnd_request.submit_request(application => 'XDO', -- Application Name
program => 'XDOREPPB', -- Program
description => 'XML Report Publisher' ,
start_time => to_char(sysdate,'YYYY/MM/DD HH24:MI:SS'), -- START_TIME
sub_request => FALSE, -- SUB_REQUEST
argument1=>'Y',
argument2=>v_request_id,
argument3=>20061,
argument4=>'WPS_Bound',
argument5=>'en',
argument6=>'N',
argument7=>'RTF',
argument8=>'PDF');
commit;
out_reqid:=v_request_id2;
return out_reqid;
dbms_output.put_line('Completed'||v_request_id||'-'||v_request_id2);
END;
Calling FND_REQUEST.SUBMIT_REQUEST From Forms
:parameter.req_id :=
FND_REQUEST.SUBMIT_REQUEST (
:blockname.appsname,
:blockname.program,
:blockname.description,
:blockname.start_time,
:blockname.sub_req = 'Y',
123, NAME_IN('ORDERS.ORDER_ID'), 'abc',
chr(0), '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '',
'', '', '', '', '', '', '', '', '', '');
IF :parameter.req_id = 0 THEN
FND_MESSAGE.RETRIEVE;
FND_MESSAGE.ERROR;
--- your more pl sql codes----
FAQs related to FND_REQUEST.SUBMIT_REQUEST API
When to use fnd_request.submit_request?
You can use this API when you want to call custom or standard concurrent programs explicitly via your custom code.
Can you call the seeded program as well?
Yes
Are all Arguments required to pass?
Yes when you care calling via Forms, In PL SQL only required parameters are required.
Why is my call to fnd_request.submit_request returning 0 ?
The call is unsuccessful.
I don’t see the submitted request in Submit Request screen?
You did not Commit in PL SQL.
Recommend Oracle doc for more information.
More articles on Oracle EBS Apps tech